Timon Harz
December 18, 2024
Networking in Swift with Alamofire
Master networking in your Swift applications with Alamofire to streamline API requests and improve app performance. Discover advanced techniques like SSL pinning, reachability monitoring, and error handling for secure and reliable communication.
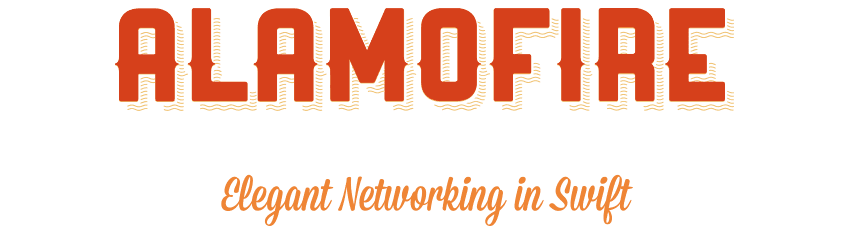
Introduction
Networking is a fundamental aspect of modern iOS applications, enabling them to communicate with remote servers to fetch data, send user inputs, and perform various online operations. In Swift, developers often utilize URLSession
for these tasks. However, while URLSession
provides a robust foundation, it can be verbose and requires manual handling of many aspects of network communication.
Alamofire, a popular Swift-based HTTP networking library, streamlines these processes by offering a higher-level abstraction. It simplifies tasks such as making network requests, handling responses, and managing errors, thereby reducing boilerplate code and enhancing code readability.
Despite these advantages, developers may encounter challenges when integrating Alamofire into their projects. For instance, Alamofire's reliance on URLSession
means that issues inherent to URLSession
, such as handling network reachability and managing background tasks, can still arise. Additionally, Alamofire's abstraction may obscure some underlying behaviors, making it more difficult to debug complex networking issues.
Moreover, Alamofire's extensive feature set can introduce additional complexity. Developers need to familiarize themselves with its API and understand how to configure it properly to suit their application's specific requirements. This learning curve can be a barrier for those new to the library or to networking in Swift in general.
In summary, while Alamofire offers a powerful and convenient approach to networking in Swift, it's essential for developers to be aware of its limitations and the challenges that may arise during its integration and use. A solid understanding of both Alamofire and the underlying networking concepts in Swift is crucial for building robust and efficient networked applications.
Alamofire is a widely adopted HTTP networking library for Swift, designed to simplify the complexities of network operations. It provides a higher-level abstraction over Apple's URLSession
, streamlining tasks such as making network requests, handling responses, and managing errors. This results in more concise and readable code compared to using URLSession
directly.
To integrate Alamofire into your Swift project, you can use dependency managers like CocoaPods or Swift Package Manager. For CocoaPods, add the following line to your Podfile
:
After running pod install
, you can import Alamofire into your Swift files:
With Alamofire integrated, performing a simple GET request becomes straightforward:
In this example, AF.request
initiates a network request to the specified URL. The validate()
method ensures that the response status code is within the acceptable range. The responseDecodable(of:)
method attempts to decode the response data into a specified type (ResponseType
in this case). The closure handles the result, allowing you to manage both success and failure scenarios effectively.
Alamofire also supports advanced features such as authentication, network reachability monitoring, and multipart form data handling. For instance, to perform a POST request with parameters:
Here, JSONEncoding.default
ensures that the parameters are encoded as JSON in the request body.
For more detailed information and advanced usage, you can refer to the official Alamofire documentation on GitHub.
By leveraging Alamofire, developers can focus more on the application's core functionality, as it abstracts away many of the complexities associated with network communication in Swift.
Setting Up Alamofire
Integrating Alamofire into your Swift project can be accomplished using various dependency managers, each offering distinct advantages. Below are the methods for incorporating Alamofire using CocoaPods, Carthage, and Swift Package Manager (SPM).
CocoaPods
Install CocoaPods: If you haven't installed CocoaPods, you can do so using the following command:
Initialize CocoaPods in Your Project: Navigate to your project directory and create a
Podfile
by running:Add Alamofire to Your Podfile: Open the
Podfile
and add Alamofire under your target:Install Alamofire: Run the following command to install Alamofire:
Open the Workspace: After installation, open the
.xcworkspace
file to work on your project.
Carthage
Install Carthage: If Carthage isn't installed, you can install it using Homebrew:
Create a Cartfile: In your project directory, create a
Cartfile
and add Alamofire:Then, add the following line to the
Cartfile
:Build Alamofire: Run the following command to build Alamofire:
Integrate Alamofire into Your Project: After building, drag the
Alamofire.framework
from theCarthage/Build/iOS
directory into your Xcode project's "Frameworks, Libraries, and Embedded Content" section.Link the Framework: Ensure that Alamofire is added to the "Embedded Binaries" section of your target settings.
Swift Package Manager (SPM)
As of now, Alamofire does not officially support integration via Swift Package Manager for iOS projects. While Alamofire builds on Linux, Windows, and Android, it is unsupported on these platforms due to missing features and issues in the underlying swift-corelibs-foundation
.
For iOS projects, it's recommended to use CocoaPods or Carthage for integrating Alamofire.
To integrate Alamofire into your Swift project, begin by importing the library at the top of your Swift files:
Alamofire provides a default Session
instance, which is typically sufficient for most networking tasks. However, for advanced configurations, you can create a custom Session
with specific settings.
To create a custom Session
, instantiate a Session
object with a SessionConfiguration
:
This custom session allows you to configure parameters such as timeout intervals and other session-specific settings.
When making network requests, you can use the session
instance to perform requests:
In this example, responseDecodable(of:)
attempts to decode the response data into a specified type (ResponseType
).
For more detailed information on configuring Alamofire and creating custom sessions, refer to the official Alamofire documentation.
Making Network Requests
To perform a GET request using Alamofire in Swift, you can utilize the AF.request
method, which simplifies network operations. Here's how you can implement it:
In this example, replace "https://api.example.com/data"
with the URL of the API endpoint you intend to access. The validate()
method ensures that the response status code is within the acceptable range. The responseDecodable(of:)
method attempts to decode the response data into a specified type (ResponseType
). The closure handles the result, allowing you to manage both success and failure scenarios effectively.
For more detailed information and advanced usage, you can refer to the official Alamofire documentation on GitHub.
To send data to a server using a POST request with Alamofire in Swift, you can follow these steps:
Define the Parameters: Create a dictionary containing the data you want to send.
Make the POST Request: Use Alamofire's
AF.request
method to send the data. Specify the HTTP method as.post
, provide the parameters, and set the encoding toJSONEncoding.default
to ensure the parameters are sent as JSON.
In this example, replace "https://httpbin.org/post"
with the URL of your API endpoint. The validate()
method ensures that the response status code is within the acceptable range. The responseJSON
method processes the response as JSON, and the closure handles both success and failure scenarios.
Advanced Networking Techniques
To handle authentication in Alamofire, you can implement Basic Authentication and OAuth 2.0 using the following approaches:
Basic Authentication
For Basic Authentication, you can manually encode the username and password into a Base64 string and include it in the Authorization
header of your request. Here's how you can do it:
In this example, replace "https://api.example.com/endpoint"
with your API endpoint. The validate()
method ensures that the response status code is within the acceptable range. The responseJSON
method processes the response as JSON, and the closure handles both success and failure scenarios.
OAuth 2.0 Authentication
Implementing OAuth 2.0 in Alamofire involves obtaining an access token and including it in the Authorization
header of your requests. Here's a basic example:
In this example, replace "https://api.example.com/endpoint"
with your API endpoint and "yourAccessToken"
with the OAuth 2.0 access token you've obtained.
For a more comprehensive implementation, especially when dealing with token expiration and refreshing, you can create a custom RequestAdapter
and RequestRetrier
to manage the authentication flow. This approach allows you to handle token expiration and refresh tokens automatically.
To monitor network reachability in your Swift application using Alamofire, you can utilize the NetworkReachabilityManager
class. This class allows you to listen for changes in network connectivity, enabling your app to respond appropriately to network status changes.
Here's how you can implement network reachability monitoring:
In this implementation, the NetworkManager
class provides a singleton instance to manage network reachability monitoring. The startMonitoring
method begins listening for network status changes, and the stopMonitoring
method stops the monitoring. The closure passed to startListening
is called whenever the network status changes, allowing you to handle different scenarios accordingly.
It's important to note that while monitoring network reachability can be useful for certain scenarios, Alamofire's NetworkReachabilityManager
is primarily designed to inform your application of network status changes. It doesn't automatically retry failed requests or manage network connectivity issues for you. Therefore, it's advisable to handle network errors gracefully within your application's networking code.
To ensure robust networking operations in Swift using Alamofire, it's essential to implement response validation, serialization, and comprehensive error handling. Here's how you can achieve this:
Response Validation
Alamofire provides the validate()
method to ensure that the response status code and content type meet your expectations. By default, validate()
checks that the status code is within the 200..<300
range and that the Content-Type
header matches the Accept
header of the request, if one is provided.
In this example, if the response status code is outside the 200..<300
range or if the content type doesn't match, Alamofire will automatically trigger the failure case.
Custom Response Validation
If you need to validate specific status codes or content types, you can customize the validation:
This approach allows you to specify exactly which status codes and content types are acceptable for your application.
Response Serialization
Alamofire offers built-in response serializers for various data types, including JSON and strings. To handle JSON responses, you can use the responseDecodable
method, which attempts to decode the response data into a specified Decodable
type:
In this example, MyData
is a struct that conforms to the Decodable
protocol. Alamofire will attempt to decode the JSON response into an instance of MyData
.
Custom Response Serialization
For more complex scenarios, you can create a custom response serializer by conforming to the DataResponseSerializerProtocol
. This allows you to define how the response data should be processed and handled:
This approach provides full control over how the response data is processed, which is useful for handling non-standard response formats or complex data structures.
Error Handling
Alamofire's AFError
provides detailed information about errors that occur during a request. You can handle errors by examining the AFError
type in the failure case:
By examining the AFError
type, you can implement more granular error handling based on the specific error encountered.
For more detailed information and advanced usage, you can refer to the official Alamofire documentation on GitHub.
Additionally, the article "Custom Response Handler in Alamofire" provides insights into creating custom response handlers to manage complex response scenarios.
By implementing these practices, you can ensure that your networking operations are robust, handle various response scenarios gracefully, and provide a seamless experience for users.
Best Practices
To enhance the reliability of your Swift application when using Alamofire for networking, it's crucial to implement effective error handling strategies. Alamofire provides robust mechanisms to manage various error scenarios, ensuring your app responds gracefully to network issues.
Custom Response Serialization
Alamofire's default response serializers handle many common scenarios, but for more complex error handling, you can create a custom response serializer by conforming to the DataResponseSerializerProtocol
. This approach allows you to define how the response data should be processed and handled, enabling you to manage both successful and error responses effectively.
In this example, CustomType
represents the type you expect to receive upon a successful response. By implementing the serialize
method, you can define how to handle the response data, including parsing and error handling. This approach provides full control over how the response data is processed, which is useful for handling non-standard response formats or complex data structures.
Request Retrying
For scenarios where network requests may fail due to transient issues, implementing a request retrier can be beneficial. Alamofire's RequestRetrier
protocol allows you to define custom retry logic, such as retrying a request upon receiving specific status codes or after a certain delay.
By implementing the should
method, you can specify the conditions under which a request should be retried, enhancing the robustness of your networking operations.
Error Handling in Response Handlers
When handling responses, it's important to distinguish between different types of errors, such as validation failures, serialization errors, and connectivity issues. Alamofire's AFError
provides detailed information about errors that occur during a request.
By examining the AFError
type, you can implement more granular error handling based on the specific error encountered, allowing for a more tailored response to different error scenarios.
To effectively monitor network reachability in your Swift application using Alamofire, you can utilize the NetworkReachabilityManager
class. This class listens for reachability changes of hosts and addresses for both cellular and WiFi network interfaces.
Implementing Network Reachability Monitoring
First, import Alamofire into your Swift file:
Next, create an instance of NetworkReachabilityManager
and start listening for network status changes:
In this setup, the closure provided to startListening
is called whenever the network reachability status changes, allowing you to respond accordingly.
Handling Network Reachability Changes
By monitoring network reachability, you can implement strategies to handle connectivity issues gracefully. For instance, you might want to pause certain operations when the network is unreachable and resume them when connectivity is restored.
To enhance the security of network communications in your Swift application using Alamofire, it's essential to implement SSL pinning and ensure proper data encryption. These practices help protect against man-in-the-middle (MITM) attacks and unauthorized data access.
Implementing SSL Pinning with Alamofire
SSL pinning ensures that your app communicates only with a trusted server by validating the server's certificate or public key. This process mitigates the risk of MITM attacks.
Obtain the Server's Certificate or Public Key:
Acquire the server's SSL certificate (typically a
.cer
or.pem
file) from your server administrator or certificate provider.Alternatively, extract the server's public key from the certificate.
Add the Certificate or Public Key to Your Project:
Import the certificate or public key into your Xcode project.
Ensure the file is included in your app’s target.
Configure Alamofire for SSL Pinning:
Create a custom
SessionDelegate
to handle the SSL pinning challenge.Override the
urlSession(_:task:didReceive:completionHandler:)
method to implement the pinning logic.
This setup ensures that Alamofire uses your custom session delegate, which handles SSL pinning during network requests.
Best Practices for SSL Pinning
Use a Trusted Certificate Authority: Always use a certificate issued by a trusted certificate authority to ensure the legitimacy of the server's certificate.
Regularly Update Certificates: Keep your certificates up to date to prevent issues related to certificate expiration and to maintain security.
Handle Certificate Rotation: Implement mechanisms to handle server certificate changes gracefully, such as updating the pinned certificates in your app when the server's certificate is updated.
Data Encryption
While SSL/TLS encrypts data in transit, it's also important to consider data encryption at rest, especially for sensitive information stored on the device.
Use Secure Storage: Utilize iOS's Keychain Services to store sensitive data securely.
Encrypt Sensitive Data: Before storing sensitive information, encrypt it using strong encryption algorithms.
By implementing SSL pinning and ensuring proper data encryption, you can significantly enhance the security of your application's network communications and protect user data from unauthorized access.
Conclusion
In this post, we've explored how Alamofire streamlines networking operations in Swift applications. By integrating Alamofire, developers can perform GET and POST requests with ease, handle authentication methods like Basic Auth and OAuth, monitor network reachability, and implement robust error handling. Additionally, we've highlighted the importance of securing network communications through practices such as SSL pinning and data encryption. By leveraging Alamofire's capabilities, developers can create efficient, secure, and reliable networking solutions for their iOS applications.
To deepen your understanding of networking in Swift with Alamofire, consider exploring the following resources:
Alamofire Tutorial with Swift (Quickstart): This tutorial introduces Alamofire, covering its capabilities in handling APIs and data feeds. Code with Chris
Alamofire iOS Advanced Techniques: This article delves into advanced Alamofire techniques, including routing and authentication cycles. Medium
Alamofire Tutorial for iOS: Advanced Usage: This tutorial explores advanced Alamofire usage, such as handling OAuth, network logging, reachability, and caching. Kodeco
Alamofire Networking in SwiftUI with Combine and MVVM: This article discusses integrating Alamofire with SwiftUI, Combine, and the MVVM pattern. Medium
Simplifying Networking in Swift using Alamofire: This article provides an overview of how Alamofire simplifies networking in Swift with practical examples. Medium
Additionally, the official Alamofire documentation offers comprehensive information on its features and usage. Alamofire
For a visual walkthrough, you might find the following video tutorial helpful:
These resources should provide a solid foundation for mastering networking in Swift using Alamofire.
Press contact
Timon Harz
oneboardhq@outlook.com
Other posts
Company
About
Blog
Careers
Press
Legal
Privacy
Terms
Security