Timon Harz
December 19, 2024
Getting Started with PencilKit: A Beginner's Guide to Drawing in iOS Apps
Learn the fundamentals of integrating PencilKit into your iOS projects and explore step-by-step instructions for implementing drawing functionalities. This guide provides the foundation you need to start creating interactive and creative applications with PencilKit.
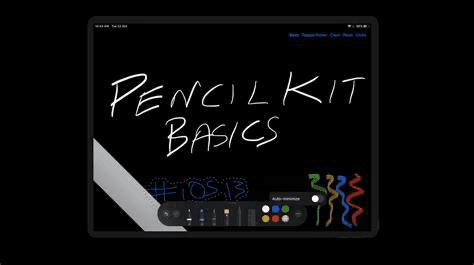
Introduction
PencilKit is Apple's robust framework designed to seamlessly integrate drawing capabilities into iOS applications. Introduced at WWDC 2019, it empowers developers to incorporate expressive, low-latency drawing experiences with minimal code. By leveraging PencilKit, apps can offer users intuitive tools for sketching, annotating, and creating digital artwork directly within the application.
At its core, PencilKit provides a suite of tools that facilitate the creation and manipulation of drawings. The PKCanvasView
serves as the primary drawing surface, allowing users to draw with precision using either an Apple Pencil or their finger. This view supports various drawing tools, including pens, markers, and highlighters, each with customizable attributes such as color and thickness. The PKToolPicker
complements the canvas by offering a user-friendly interface for selecting and customizing these tools, enhancing the overall drawing experience.
One of the standout features of PencilKit is its responsiveness and low latency, ensuring that user input is captured and rendered in real-time. This responsiveness is crucial for applications that require precise and fluid drawing interactions, such as note-taking apps, design tools, and educational platforms. The framework's integration with Apple's hardware, including the Apple Pencil, further enhances its performance, providing pressure sensitivity, tilt recognition, and other advanced features that contribute to a natural and intuitive drawing experience.
Beyond basic drawing functionalities, PencilKit offers advanced capabilities for managing and manipulating drawings. Developers can access the underlying data of a drawing through the PKDrawing
class, which represents the collection of strokes and tools used. This access allows for features such as undo and redo operations, saving and loading drawings, and even performing machine learning-based analysis on the drawings. For instance, developers can inspect, modify, and construct PencilKit drawings to build features that utilize recognition and modification, enabling a wide range of creative possibilities within their applications.
PencilKit's versatility extends to its compatibility across Apple's ecosystem. While it is optimized for iPadOS, particularly for devices equipped with Apple Pencil, the framework also supports iOS and macOS, allowing developers to create cross-platform drawing experiences. This cross-platform support ensures that applications can reach a broader audience, providing consistent and high-quality drawing functionalities regardless of the device being used.
Integrating drawing features into iOS applications has become a pivotal strategy for developers aiming to enhance user engagement and satisfaction. In an era where user experience is paramount, the ability to offer interactive and creative functionalities can significantly differentiate an app in a crowded marketplace.
Drawing capabilities empower users to express themselves, annotate content, and interact with the app in a more personalized manner. This level of interactivity fosters a deeper connection between the user and the application, leading to increased engagement. For instance, apps that allow users to sketch, highlight, or annotate images and documents provide a sense of ownership and creativity, which can enhance user satisfaction and retention.
Moreover, incorporating drawing features can transform passive users into active participants. When users are given tools to create or modify content, they are more likely to spend additional time within the app, exploring its functionalities and features. This active engagement not only boosts the time spent on the app but also increases the likelihood of users returning, thereby improving retention rates.
From a business perspective, enhancing user engagement through interactive features like drawing can lead to higher conversion rates. Engaged users are more inclined to explore premium features, make in-app purchases, or share the app with others, thereby contributing to the app's growth and profitability. For example, a note-taking app that allows users to draw and annotate can encourage users to upgrade to a premium version for additional features, directly impacting revenue.
Furthermore, drawing features can serve as a unique selling point, setting an app apart from competitors. In a saturated market, offering innovative functionalities can attract new users and retain existing ones. Apps that provide creative tools often receive positive reviews and word-of-mouth recommendations, which can enhance the app's reputation and visibility in the App Store.
Incorporating drawing functionalities also aligns with the growing trend of personalization in app development. Users increasingly seek applications that cater to their individual preferences and allow them to customize their experience. By enabling users to create and modify content, developers can meet this demand, leading to higher user satisfaction and loyalty.
Prerequisites
To develop applications utilizing PencilKit, Apple's framework for integrating drawing capabilities into iOS apps, certain system requirements must be met. PencilKit is available starting from iOS 13.0 and requires Xcode 11 or later.
When setting up your development environment, ensure that your macOS system supports the latest version of Xcode. Xcode 11 introduced significant enhancements, including support for Swift 5 and the integration of new frameworks like PencilKit. To check your macOS version, navigate to the Apple menu and select "About This Mac." If your macOS version is compatible, you can download Xcode 11 from the Mac App Store.
After installing Xcode 11, you can create a new project targeting iOS 13.0 or later. In your project's settings, set the deployment target to iOS 13.0 to ensure compatibility with devices running this version or newer.
To incorporate PencilKit into your project, import the framework at the beginning of your Swift files:
This import statement grants access to the classes and methods provided by PencilKit, such as PKCanvasView
for drawing surfaces and PKToolPicker
for tool selection.
Here's an example of how to set up a basic drawing canvas using PKCanvasView
:
In this example, PKCanvasView
is initialized with the view's bounds and added as a subview, allowing users to draw within the view controller's view.
For more detailed information and advanced configurations, refer to Apple's official PencilKit documentation.
PencilKit, Apple's framework for integrating drawing capabilities into iOS applications, is designed to provide a seamless and responsive drawing experience across a range of devices. While it is optimized for use with Apple Pencil on iPads, it also supports finger input on iPhones, ensuring that users can engage with drawing features regardless of the device they are using.
On iPads, PencilKit leverages the precision and pressure sensitivity of the Apple Pencil, offering a natural and intuitive drawing experience. The Apple Pencil's capabilities, such as tilt recognition and varying pressure levels, are fully supported, allowing for detailed and expressive drawings. This integration is particularly beneficial for applications focused on design, illustration, and note-taking, where precision and responsiveness are crucial.
For iPhone users, PencilKit adapts to support finger input, ensuring that drawing functionalities are accessible even without specialized hardware. While the experience may not offer the same level of precision as the Apple Pencil, it still provides a responsive and engaging drawing environment. This flexibility allows developers to create applications that cater to a broader audience, accommodating users who may not have access to an iPad or Apple Pencil.
To implement drawing capabilities that support both Apple Pencil and finger input, developers can configure the drawingPolicy
property of the PKCanvasView
to .anyInput
. This setting enables the canvas to accept input from any source, including both the Apple Pencil and the user's finger. Here's an example of how to set this up in Swift:
In this example, the PKCanvasView
is configured to accept input from any source, ensuring that users can draw using either the Apple Pencil or their finger, depending on the device they are using.
It's important to note that while PencilKit provides a robust drawing environment, the experience may vary between devices. On iPads with Apple Pencil, users can take advantage of advanced features such as pressure sensitivity and tilt recognition, which are not available when using finger input on iPhones. Therefore, developers should consider these differences when designing their applications to ensure a consistent and satisfying user experience across all supported devices.
By understanding and leveraging the hardware capabilities of the devices, developers can create applications that offer a rich and engaging drawing experience, whether the user is on an iPad with Apple Pencil or an iPhone using their finger.
Setting Up Your Development Environment
To develop applications utilizing PencilKit, Apple's framework for integrating drawing capabilities into iOS applications, it's essential to have the latest version of Xcode installed on your Mac. Xcode is Apple's integrated development environment (IDE) that provides all the tools necessary for app development across various Apple platforms, including iOS, macOS, watchOS, and tvOS.
Downloading and Installing Xcode via the Mac App Store
The most straightforward method to download and install Xcode is through the Mac App Store. This approach ensures that you receive the latest stable version of Xcode, complete with all the necessary tools and updates.
Open the Mac App Store: Click on the App Store icon located in your Dock or find it using Spotlight search.
Sign In: If you're not already signed in, click on the "Sign In" button at the bottom left corner and enter your Apple ID credentials.
Search for Xcode: Use the search bar at the top left corner of the App Store window to type in "Xcode."
Download and Install: Locate Xcode in the search results, click on the "Get" button, and then click "Install." You may be prompted to enter your Apple ID password to authorize the download.
Wait for the Installation to Complete: The download size for Xcode is substantial (approximately 8 GB), so the process may take some time depending on your internet connection speed. Once the download is complete, the installation will proceed automatically.
Launch Xcode: After installation, you can find Xcode in your Applications folder. Open it to begin setting up your development environment.
Downloading and Installing Xcode from the Apple Developer Website
Alternatively, you can download Xcode directly from Apple's Developer website, which allows you to access specific versions of Xcode, including beta releases or older versions.
Visit the Apple Developer Downloads Page: Navigate to the Apple Developer Downloads page.
Sign In: Click on the "Sign In" button and enter your Apple ID credentials. If you don't have an Apple ID, you'll need to create one.
Locate Xcode: In the search bar, type "Xcode" to filter the results.
Select the Desired Version: Choose the version of Xcode you wish to download. For the latest stable release, select the most recent version.
Download the Xcode Archive: Click on the download link for the
.xip
file corresponding to the selected Xcode version.Extract the Xcode Archive: Once the download is complete, locate the
.xip
file in your Downloads folder and double-click it. This will extract the Xcode application.Move Xcode to the Applications Folder: Drag the extracted Xcode application into your Applications folder to complete the installation.
Launch Xcode: Open Xcode from the Applications folder to begin setting up your development environment.
Installing Command Line Tools
In addition to the Xcode IDE, you may need to install the Xcode Command Line Tools, which provide essential tools for software development, such as compilers and debuggers.
Open Terminal: Navigate to Applications > Utilities > Terminal.
Install Command Line Tools: Enter the following command and press Enter:
Follow the On-Screen Instructions: A prompt will appear asking if you want to install the command line developer tools. Click "Install" to proceed.
Wait for the Installation to Complete: The installation process will take a few minutes. Once completed, the command line tools will be ready for use.
Verifying the Installation
After installing Xcode and the command line tools, it's important to verify that everything is set up correctly.
Verify Xcode Installation: Open Terminal and enter the following command:
This command should return the path to the Xcode application, indicating that Xcode is installed correctly.
Verify Command Line Tools Installation: In Terminal, enter:
This command should display the version of the GCC compiler, confirming that the command line tools are installed.
Updating Xcode
To ensure you have the latest features and security updates, it's important to keep Xcode updated.
Via the Mac App Store: If you installed Xcode through the Mac App Store, updates will be available in the "Updates" tab. You can also enable automatic updates in the App Store preferences.
Via the Developer Website: If you downloaded Xcode from the Apple Developer website, you'll need to manually download and install the latest version from the Apple Developer Downloads page.
By following these steps, you can successfully download, install, and set up Xcode on your Mac, providing you with the necessary tools to develop applications using PencilKit and other Apple frameworks.
To begin developing an iOS application using PencilKit, it's essential to set up a new project in Xcode. This process involves creating a new workspace, configuring project settings, and preparing the environment for iOS development.
Launching Xcode
Start by opening Xcode on your Mac. If Xcode is not already installed, you can download it from the Mac App Store. Once installed, locate the Xcode icon in your Applications folder and double-click to launch it.
Creating a New Project
Upon launching Xcode, you'll be greeted with the welcome window. Click on "Create a new Xcode project" to initiate the project creation process. Alternatively, you can navigate to the menu bar and select File
> New
> Project
.
Selecting a Template
Xcode offers various templates tailored for different types of applications. For an iOS app, select the "App" template under the iOS section. This template provides a basic structure for building iOS applications. Click "Next" to proceed.
Configuring Project Details
In the next window, you'll need to provide essential information about your project:
Product Name: Enter the name of your application.
Team: If you have an Apple Developer account, select your team from the dropdown. If not, you can choose "None" for now.
Organization Name: Specify your organization or personal name.
Organization Identifier: Typically in reverse domain name format (e.g., com.example).
Bundle Identifier: This is automatically generated based on the product name and organization identifier.
Language: Choose "Swift" as the programming language.
User Interface: Select "SwiftUI" for a declarative UI framework or "Storyboard" for a visual interface builder.
Use Core Data: Check this box if your app requires a local database.
Include Tests: Decide if you want to include unit and UI tests in your project.
After filling in these details, click "Next."
Choosing a Save Location
Select a directory on your Mac where you want to save the project. It's advisable to choose a location that's easy to access and organize. After selecting the location, click "Create" to generate your new project.
Exploring the Project Structure
Once the project is created, Xcode will display the main workspace window. Here, you'll find several key components:
Project Navigator: Located on the left, it displays all the files and folders in your project.
Editor Area: In the center, this is where you'll write and edit your code.
Canvas: If using SwiftUI, the canvas allows you to see a live preview of your UI.
Inspector Area: On the right, it provides details and settings for the selected file or object.
Setting Up the User Interface
If you chose "Storyboard" as your user interface option, you can begin designing your app's UI by opening the Main.storyboard
file. Here, you can drag and drop UI elements like buttons, labels, and text fields to construct your app's interface.
For a SwiftUI-based project, open the ContentView.swift
file. SwiftUI allows you to build UIs using a declarative syntax. You can add UI components directly in the code, and the canvas will display a live preview of your design.
Adding PencilKit to Your Project
To incorporate PencilKit into your project, import the framework at the top of your Swift files:
This import statement grants access to the classes and methods provided by PencilKit, such as PKCanvasView
for drawing surfaces and PKToolPicker
for tool selection.
Here's an example of how to set up a basic drawing canvas using PKCanvasView
:
In this example, PKCanvasView
is initialized with the view's bounds and added as a subview, allowing users to draw within the view controller's view.
Running Your Application
To test your application, select a simulator or a connected device from the toolbar's device selection menu. Then, click the "Run" button (a play icon) or press Cmd + R
to build and run your app. Xcode will compile your code, launch the simulator or deploy to the device, and start your application.
By following these steps, you've successfully created a new iOS project in Xcode, set up the user interface, integrated PencilKit, and run your application. This foundation allows you to build and expand your app's functionality as needed.
Integrating PencilKit into Your App
To integrate PencilKit into your iOS project, you need to import the framework into your Swift files. This allows you to access the classes and methods provided by PencilKit, such as PKCanvasView
for drawing surfaces and PKToolPicker
for tool selection.
Importing PencilKit
At the top of your Swift file, add the following import statement:
This line grants your file access to the PencilKit framework, enabling you to utilize its drawing capabilities.
Setting Up the Drawing Canvas
After importing PencilKit, you can set up a drawing canvas using PKCanvasView
. Here's an example of how to initialize and configure a basic drawing canvas:
In this example, PKCanvasView
is initialized with the view's bounds and added as a subview. The drawingPolicy
is set to .anyInput
to allow both Apple Pencil and finger input. The background color is set to white, and the initial drawing tool is configured as a black pen with a width of 10.
Adding a Tool Picker
To provide users with a selection of drawing tools, you can add a PKToolPicker
to your view. Here's how to implement it:
In this setup, the PKToolPicker
is initialized and configured to be visible for the canvasView
. The canvasView
is added as an observer to the toolPicker
, and it becomes the first responder to activate the tool picker.
Handling Drawing Changes
To respond to changes in the drawing, you can implement the PKCanvasViewDelegate
protocol. Here's how to set it up:
By conforming to the PKCanvasViewDelegate
protocol and implementing the canvasViewDrawingDidChange(_:)
method, you can respond to changes in the drawing. In this example, a simple print statement is used to indicate when the drawing has changed.
By following these steps, you can effectively import and utilize the PencilKit framework in your iOS project, enabling you to add drawing capabilities to your application.
Integrating a drawing canvas into your iOS application using PencilKit involves adding a PKCanvasView
to your view controller. This view captures user input from Apple Pencil or touch gestures and displays the resulting drawings.
Adding a PKCanvasView Programmatically
To add a PKCanvasView
programmatically, follow these steps:
Import the PencilKit Framework: At the top of your Swift file, import the PencilKit framework to access its classes and methods.
Declare a PKCanvasView Property: In your view controller class, declare a property for the
PKCanvasView
.In this example,
PKCanvasView
is initialized with the view's bounds and added as a subview. ThedrawingPolicy
is set to.anyInput
to allow both Apple Pencil and finger input. The background color is set to white, and the initial drawing tool is configured as a black pen with a width of 10.
Adding a PKCanvasView Using Interface Builder
Alternatively, you can add a PKCanvasView
using Interface Builder:
Add a UIView to Your Storyboard: Drag a
UIView
onto your view controller's scene in the storyboard.Set the Custom Class: In the Identity Inspector, set the custom class of the
UIView
toPKCanvasView
.Create an Outlet: Control-drag from the
PKCanvasView
in the storyboard to your view controller class to create an IBOutlet.Configure the Canvas View: In your view controller's
viewDidLoad
method, configure thePKCanvasView
as needed.
Handling Drawing Changes
To respond to changes in the drawing, implement the PKCanvasViewDelegate
protocol:
Conform to PKCanvasViewDelegate: In your view controller class, conform to the
PKCanvasViewDelegate
protocol.Implement Delegate Methods: Implement the delegate methods to handle drawing changes.
Set the Delegate: Assign the delegate property of the
PKCanvasView
to your view controller.
By following these steps, you can effectively add a PKCanvasView
to your view controller, allowing users to draw within your application.
Integrating a PKToolPicker
into your iOS application enhances the drawing experience by providing users with a selection of drawing tools, colors, and additional options. The PKToolPicker
is a draggable palette that displays these tools, allowing users to choose their preferred drawing instrument.
Understanding PKToolPicker
The PKToolPicker
manages a palette that includes various drawing tools such as pens, pencils, and erasers, along with color options. It is designed to be used in conjunction with a PKCanvasView
, which serves as the drawing surface. By integrating the PKToolPicker
, you provide users with an intuitive interface to select and customize their drawing tools.
Setting Up PKToolPicker
To configure the PKToolPicker
in your view controller, follow these steps:
Import the PencilKit Framework: Ensure that the PencilKit framework is imported at the top of your Swift file.
Declare a PKToolPicker Property: In your view controller class, declare a property for the
PKToolPicker
.In this setup, the
PKToolPicker
is initialized and configured to be visible for thecanvasView
. ThecanvasView
is added as an observer to thetoolPicker
, and it becomes the first responder to activate the tool picker.
Customizing the Tool Picker
The PKToolPicker
can be customized to suit the needs of your application. For instance, you can set the initial drawing tool, adjust the visibility of the tool picker, and manage its position on the screen.
To set the initial drawing tool, you can assign a PKInkingTool
to the tool
property of the canvasView
:
This line sets the initial drawing tool to a black pen with a width of 10.
To adjust the visibility of the tool picker, you can use the setVisible(_:forFirstResponder:)
method:
This line makes the tool picker visible when the canvasView
becomes the first responder.
To manage the position of the tool picker, you can use the setFrame(_:)
method:
This line sets the position and size of the tool picker on the screen.
Handling Tool Picker Visibility
The visibility of the PKToolPicker
is managed based on the first responder status of the PKCanvasView
. By making the canvasView
the first responder, you activate the tool picker, allowing users to select drawing tools.
To make the canvasView
the first responder, use the becomeFirstResponder()
method:
This line activates the tool picker, enabling users to choose their preferred drawing tools.
By following these steps, you can effectively integrate and configure the PKToolPicker
in your iOS application, providing users with a rich and interactive drawing experience.
Implementing Drawing Features
Capturing and displaying user drawings in your iOS application using PencilKit involves setting up a PKCanvasView
to handle touch inputs and render the resulting drawings. This process enables users to create and view drawings directly within your app.
Setting Up the Drawing Environment
To begin, ensure that the PencilKit framework is imported into your project:
Next, declare a PKCanvasView
property in your view controller:
In this setup, the PKCanvasView
is initialized to fill the view's bounds, and its properties are configured to accept input from both Apple Pencil and touch gestures.
Handling User Input
The PKCanvasView
automatically captures touch inputs, including those from Apple Pencil and fingers, and renders the corresponding strokes on the canvas. To respond to changes in the drawing, implement the PKCanvasViewDelegate
protocol:
By conforming to the PKCanvasViewDelegate
protocol and implementing the canvasViewDrawingDidChange(_:)
method, you can execute custom actions whenever the drawing changes.
Saving and Loading Drawings
To persist user drawings, you can save the drawing data to a file and load it when needed.
Saving the Drawing:
Loading the Drawing:
These methods allow you to save the drawing data to a file or database and load it back into the PKCanvasView
when needed.
Clearing the Canvas
To provide users with the ability to clear the canvas, you can implement a method to reset the drawing:
This method resets the drawing to an empty state, effectively clearing the canvas.
By following these steps, you can effectively capture and display user drawings in your iOS application using PencilKit, providing a responsive and interactive drawing experience.
Saving user drawings created with PencilKit in your iOS application is essential for preserving user-generated content. PencilKit provides robust methods to save drawings either as image files or as data representations, allowing for flexible storage and retrieval.
Saving Drawings as Images
To save a drawing as an image, you can render the drawing from the PKCanvasView
into a UIImage
. This approach is particularly useful when you need to display the drawing as an image or share it with other applications.
Here's how you can convert the drawing to a UIImage
:
In this function, drawing.image(from:scale:)
generates a UIImage
from the drawing's bounds at the specified scale. The scale factor determines the resolution of the resulting image; a higher scale factor results in a higher-resolution image.
Once you have the UIImage
, you can save it to the photo library, share it, or store it in a file. For example, to save the image to the photo library:
Saving Drawings as Data
Alternatively, you can save the drawing as data, which is useful for storing the drawing in a database or sending it over a network. PencilKit's PKDrawing
class conforms to the NSSecureCoding
protocol, allowing it to be serialized and deserialized.
To save the drawing as data:
In this function, drawing.dataRepresentation()
converts the PKDrawing
object into a Data
object. This data can then be saved to a file, stored in a database, or transmitted over a network.
To load the drawing from data:
This function attempts to create a PKDrawing
from the provided data and assigns it to the canvasView
.
Considerations
Data Persistence: When saving drawings as data, ensure that the storage medium supports the data's size and format. For instance, Core Data can store
Data
objects, but you should consider the potential size of the drawing data and the performance implications.Error Handling: The methods provided above use optional returns and do not include error handling. In a production environment, you should handle potential errors appropriately, such as by using
do-catch
blocks or propagating errors to the caller.Performance: Converting drawings to images at high resolutions can be resource-intensive. It's advisable to balance the resolution with performance requirements, especially if the drawings are large or if the application runs on devices with limited resources.
By utilizing these methods, you can effectively save and manage user drawings in your iOS application, providing a seamless experience for users to create, store, and share their content.
Implementing a function to clear the drawing area in your iOS application using PencilKit is straightforward. The PKCanvasView
class provides a drawing
property that represents the current drawing on the canvas. To clear the canvas, you can assign a new, empty PKDrawing
to this property.
Here's how you can implement a function to clear the canvas:
In this function, PKDrawing()
initializes a new, empty drawing, effectively clearing any existing content on the canvas.
To provide users with a way to clear the canvas, you can add a "Clear" button to your user interface and connect it to the clearCanvas
function. For example, if you're using UIKit, you can add a UIButton and set its action to call clearCanvas
when tapped.
This setup allows users to clear the canvas by tapping the "Clear" button.
It's important to note that clearing the canvas in this manner removes all drawing content. If you need to implement undo and redo functionality, you should utilize the undoManager
property of your view controller to manage drawing states. This approach enables users to revert to previous drawings or redo actions as needed.
Advanced Features
Implementing undo and redo functionality in your iOS application using PencilKit enhances the user experience by allowing users to revert and reapply changes to their drawings. While the PKToolPicker
provides built-in undo and redo controls on iPads, these controls are not available on iPhones. Therefore, you need to implement custom undo and redo actions to manage the drawing history effectively.
Understanding the Undo Manager
iOS provides the UndoManager
class, which manages a stack of undo and redo operations. By integrating UndoManager
with your PKCanvasView
, you can track drawing changes and enable undo and redo actions.
Integrating UndoManager with PKCanvasView
To implement undo and redo functionality, you can utilize the UndoManager
in conjunction with the PKCanvasView
. Here's how you can set it up:
In this setup, the canvasViewDrawingDidChange(_:)
delegate method is implemented to register undo operations whenever the drawing changes. The registerUndo(withTarget:handler:)
method of UndoManager
is used to register the current drawing state, allowing it to be reverted when the undo action is triggered.
Handling Undo and Redo Actions
To handle undo and redo actions, you can add buttons to your user interface and connect them to the undo()
and redo()
methods:
These methods invoke the corresponding UndoManager
methods to perform the undo and redo operations.
Considerations
Undo Manager Scope: The
UndoManager
instance should be retained for the duration of the drawing session to maintain the undo and redo history.Performance: Be mindful of the performance implications when registering undo operations, especially for large drawings. It's advisable to register undo operations at appropriate intervals to balance performance and functionality.
User Interface: Ensure that the undo and redo buttons are enabled or disabled appropriately based on the availability of actions in the undo and redo stacks.
By integrating the UndoManager
with your PKCanvasView
, you can provide robust undo and redo functionality, enhancing the interactivity and usability of your drawing application.
Customizing drawing tools in your iOS application using PencilKit allows you to tailor the drawing experience to your specific requirements. PencilKit provides a set of predefined tools, but you can create custom tools or modify existing ones to enhance functionality and user engagement.
Creating Custom Drawing Tools
In iOS 18 and later, PencilKit introduced the ability to create completely custom tools within the tool picker. This enhancement enables you to define unique drawing behaviors and appearances.
To create a custom drawing tool, you can subclass PKTool
and implement the necessary methods to define the tool's behavior. Here's an example of how to create a custom tool:
In this example, CustomDrawingTool
subclasses PKInkingTool
, which is a subclass of PKTool
. You can override methods and properties to customize the tool's behavior, such as its appearance, stroke width, and color.
Adding Custom Tools to the Tool Picker
Once you've created a custom drawing tool, you can add it to the PKToolPicker
to make it available for selection. The PKToolPicker
is a UI component that allows users to choose different drawing tools.
To add your custom tool to the tool picker, you can use the setVisible(_:forFirstResponder:)
method to make the tool picker visible and set the first responder. Then, you can add your custom tool to the tool picker using the setTools(_:for:)
method.
In this setup, the setupToolPicker
method initializes the PKToolPicker
, makes it visible, and adds the custom tool to the tool picker. The setTools(_:for:)
method associates the custom tool with the drawing tool type.
Modifying Existing Tools
If you prefer to modify existing tools rather than creating new ones, you can customize the properties of the predefined PKInkingTool
or other tool classes. For example, to create a custom pen tool with a specific color and width:
This creates a blue pen tool with a width of 10 points. You can then add this custom tool to the tool picker as shown in the previous example.
Considerations
Tool Behavior: When creating custom tools, consider how they interact with the canvas. You may need to override methods to define the tool's behavior during drawing, such as pressure sensitivity or tilt response.
User Interface: Ensure that the custom tools are clearly represented in the tool picker, allowing users to easily identify and select them. You can customize the appearance of the tool picker to match your application's design.
Performance: Custom tools can introduce additional complexity. Test the performance of your custom tools to ensure they provide a smooth and responsive drawing experience.
By creating and customizing drawing tools, you can provide a tailored drawing experience that aligns with your application's unique requirements. For more detailed information on customizing drawing tools with PencilKit, refer to Apple's official documentation.
Managing multiple drawings within an iOS application using PencilKit involves creating a system that allows users to create, save, retrieve, and manipulate multiple drawings seamlessly. PencilKit's PKDrawing
class serves as the core data model for individual drawings, encapsulating the strokes and attributes of a drawing. To handle multiple drawings, you'll need to implement a storage solution, such as Core Data or a file-based approach, to persist and manage these drawings.
Creating and Managing Multiple Drawings
Each drawing in PencilKit is represented by a PKDrawing
object, which contains an array of PKStroke
objects. To manage multiple drawings, you can create a collection of PKDrawing
instances, each corresponding to a separate drawing.
Here's an example of how to create a new drawing and add it to a collection:
In this example, DrawingManager
maintains an array of PKDrawing
objects. The createNewDrawing
method initializes a new drawing and adds it to the collection. The getDrawing(at:)
method retrieves a drawing at a specified index, and the deleteDrawing(at:)
method removes a drawing from the collection.
Persisting Drawings
To ensure that drawings persist between app launches, you need to save them to a storage medium. Core Data is a robust framework for managing model layer objects in an application, and it integrates well with PencilKit.
Here's how you can save and retrieve PKDrawing
objects using Core Data:
In this implementation, DrawingEntity
is a Core Data entity with a data
attribute of type Data
to store the serialized PKDrawing
. The createNewDrawing
method creates a new DrawingEntity
, serializes an empty PKDrawing
, and saves it to the context. The getDrawing(at:)
method retrieves a drawing by deserializing the stored data. The deleteDrawing(at:)
method removes a drawing from the context.
Handling Drawing Selection and Display
To display and edit a selected drawing, you can implement a method to set the current drawing in your view controller:
In this example, setDrawing(_:)
sets the currentDrawing
and updates the PKCanvasView
to display the selected drawing.
Considerations
Concurrency: When managing multiple drawings, especially in a multi-threaded environment, ensure that access to shared resources is properly synchronized to prevent data corruption.
Error Handling: Implement robust error handling for operations like saving and retrieving drawings to handle scenarios such as data corruption or storage failures gracefully.
User Interface: Provide a user-friendly interface for selecting, creating, and deleting drawings. Consider implementing features like previews, sorting, and categorization to enhance the user experience.
By effectively managing multiple drawings, you can create a dynamic and interactive drawing application that allows users to create, save, and manipulate multiple drawings seamlessly.
Testing and Debugging
When developing applications that utilize PencilKit, especially those incorporating Apple Pencil features, it's imperative to conduct testing on physical devices. While the iOS Simulator offers a convenient environment for initial development and testing, it does not fully replicate the nuanced behaviors and performance characteristics of actual hardware. This discrepancy is particularly evident when dealing with input methods like the Apple Pencil, which offers unique capabilities such as pressure sensitivity, tilt recognition, and low-latency input.
The iOS Simulator does not currently support simulating Apple Pencil input.
This limitation means that developers cannot accurately assess how their applications will respond to Apple Pencil interactions within the Simulator. Features like pressure sensitivity, tilt, azimuth, and altitude angles, which are integral to the Apple Pencil experience, cannot be effectively tested in the Simulator environment.
Testing on a physical device equipped with an Apple Pencil ensures that developers can evaluate the application's performance and responsiveness under real-world conditions. This approach allows for the assessment of latency, precision, and the overall user experience, which are critical for applications that rely on detailed drawing or annotation functionalities.
Moreover, physical device testing enables developers to observe how the application handles various input scenarios, such as rapid strokes, varying pressure levels, and different tilt angles. These factors can significantly influence the application's behavior and performance, and only through testing on actual hardware can developers gain a comprehensive understanding of how their application will perform in the hands of end-users.
Incorporating physical device testing into the development workflow is essential for ensuring that applications deliver a seamless and intuitive experience for users. By leveraging the full capabilities of the Apple Pencil and conducting thorough testing on physical devices, developers can create applications that meet the high standards expected by users and provide a robust and engaging experience.
When developing applications that utilize PencilKit, it's essential to implement effective debugging strategies to identify and resolve issues related to drawing functionalities. Common challenges include unresponsive drawing areas, unexpected behaviors during drawing, and integration issues with other components. Addressing these issues requires a systematic approach to debugging.
1. Verifying Canvas Configuration
Ensure that your PKCanvasView
is properly configured and added to the view hierarchy. A common mistake is neglecting to set the drawingPolicy
property, which determines the input method. By default, drawingPolicy
is set to .pencilOnly
, meaning the canvas will only respond to Apple Pencil input. If you intend to allow drawing with both Apple Pencil and finger input, set the drawingPolicy
to .anyInput
.
This configuration ensures that the canvas responds to both Apple Pencil and finger inputs.
2. Inspecting Constraints and Layout
Layout issues can prevent the PKCanvasView
from displaying correctly. Use Xcode's "Debug View Hierarchy" feature to inspect the view hierarchy and identify any constraints that might be causing layout problems. For instance, if you encounter errors like "unable to simultaneously satisfy constraints," it indicates conflicting constraints that need to be resolved. Review each constraint to identify and remove any unwanted ones.
3. Handling Tiling Issues
The PKCanvasView
internally uses tiling to manage large drawings efficiently. If you notice unexpected tiling behavior or performance issues, consider adjusting the drawing
property to a smaller area or optimizing the drawing content. Be aware that PKCanvasView
does not natively support tiling across multiple canvases, so implementing custom tiling solutions may require additional development effort.
4. Debugging Drawing Responsiveness
If the drawing area becomes unresponsive, ensure that the PKCanvasView
is properly initialized and added to the view hierarchy. Check that the drawingPolicy
is set correctly and that there are no conflicting gestures or touch event handlers intercepting the drawing input.
5. Utilizing Logging and Breakpoints
Implement logging statements to track the flow of execution and identify where issues occur. Set breakpoints in your code to inspect the state of variables and the view hierarchy at runtime. This approach helps in pinpointing the exact location of the problem.
6. Reviewing Documentation and Community Resources
Consult the official Apple Developer Documentation for PencilKit
to understand its intended usage and limitations. Engage with developer communities, such as Stack Overflow and the Apple Developer Forums, to seek advice and solutions from other developers who may have encountered similar issues.
By systematically applying these debugging strategies, you can effectively identify and resolve issues related to PencilKit in your application, ensuring a smooth and responsive drawing experience for users.
Conclusion
PencilKit is Apple's robust framework that enables developers to integrate drawing capabilities into iOS applications, providing users with a seamless and responsive drawing experience. By leveraging PencilKit, developers can create applications that support both Apple Pencil and finger input, allowing for a wide range of creative possibilities.
To begin utilizing PencilKit, ensure that your development environment meets the necessary system requirements. PencilKit requires Xcode 11 and iOS 13 or later. This ensures compatibility with the latest features and optimizations provided by the framework.
While PencilKit is optimized for Apple Pencil on iPads, it also supports finger input on iPhones. This versatility allows developers to reach a broader audience, accommodating users across different device types.
To start integrating PencilKit into your project, download and install the latest version of Xcode from the Mac App Store. Once installed, create a new iOS project and import the PencilKit framework by adding the following line to your Swift file:
Next, add a PKCanvasView
to your view controller's view. This can be done programmatically or by dragging a PKCanvasView
object onto your storyboard. Ensure that the canvas view is properly constrained within your layout to provide an optimal drawing area.
To enable users to select different drawing tools, configure a PKToolPicker
. This tool picker provides a user-friendly interface for selecting tools such as pens, pencils, and erasers. You can set up the tool picker as follows:
This setup ensures that the tool picker is visible and associated with the canvas view, allowing users to choose their desired drawing tools.
To capture and display user drawings, implement the PKCanvasViewDelegate
protocol in your view controller. This protocol provides methods to respond to drawing events, such as when a drawing is completed or when the drawing changes. For example, to handle the completion of a drawing, you can implement the following delegate method:
To save drawings, you can convert the PKDrawing
object to an image or data. For instance, to convert the drawing to a UIImage, you can use the following code:
This code captures the current drawing as an image, which can then be saved to the device's photo library or shared with other applications.
To implement a clear function that resets the drawing area, you can use the following code:
This line of code clears the current drawing, providing users with a fresh canvas to work on.
Implementing undo and redo functionality enhances the user experience by allowing users to revert or reapply changes. PencilKit provides built-in support for undo and redo operations. To enable this functionality, ensure that the PKCanvasView
is the first responder and that the tool picker is properly configured. The undo and redo actions can be triggered using the standard undo and redo gestures provided by the system.
Customizing drawing tools allows developers to create unique drawing experiences tailored to their application's needs. PencilKit supports the creation of custom tools by subclassing PKTool
and implementing the desired behavior. For example, to create a custom stamp tool, you can subclass PKTool
and override the necessary methods to define the tool's behavior and appearance.
Managing multiple drawings within an app can be achieved by maintaining separate instances of PKDrawing
objects. You can create a new drawing by initializing a PKDrawing
object and assigning it to the drawing
property of the PKCanvasView
. This approach allows users to work on multiple drawings simultaneously or switch between different drawings as needed.
Testing your application on physical devices, especially those equipped with Apple Pencil, is crucial for ensuring that the drawing features function as intended. While the iOS Simulator is a valuable tool for initial development, it does not fully replicate the performance and input characteristics of actual hardware. Testing on physical devices allows you to assess the responsiveness, accuracy, and overall user experience of the drawing features.
In summary, integrating PencilKit into your iOS application enables you to provide users with a rich and interactive drawing experience. By following the steps outlined above and leveraging the capabilities of PencilKit, you can create applications that support a wide range of drawing functionalities, from basic sketches to complex annotations. Remember to test your application on physical devices to ensure optimal performance and user satisfaction.
Exploring and experimenting with PencilKit can significantly enhance the drawing capabilities of your iOS applications, providing users with a rich and interactive experience. By integrating PencilKit, you can enable features such as sketching, annotating, and creating digital artwork directly within your app.
To begin, familiarize yourself with the basics of PencilKit by reviewing Apple's official documentation. This resource offers comprehensive information on setting up and utilizing the framework effectively.
For a practical demonstration, consider watching the "PencilKit: Basics & Intro (iOS)" tutorial. This video provides a hands-on approach to implementing drawing functionalities in your app.
Additionally, the article "PencilKit Basics — The Drawing Environment For Your iOS App" offers insights into creating a basic PencilKit example, including adding a PKCanvasView
to your project and implementing the PKCanvasViewDelegate
protocol.
By leveraging these resources and experimenting with PencilKit's features, you can create engaging and interactive drawing experiences that will captivate your users. Don't hesitate to explore the various capabilities of PencilKit to discover how it can best serve the needs of your application.
Press contact
Timon Harz
oneboardhq@outlook.com
Other posts
Company
About
Blog
Careers
Press
Legal
Privacy
Terms
Security